具有删除功能的反应模式 [英] React modal with delete functionality
问题描述
我有一个应用程序,它显示行中的项目列表.每个项目都有一个相应的删除按钮.当我单击它时,它会显示一个带有确认的模式.当我单击取消"时,该操作被取消.当我单击确认"时,模式如下:
1. 它显示一个加载图标
2. 它改变了样式(颜色和文字 - 现在它说它完成了)
3.它删除项目
到目前为止,我得到的只是显示模式,与本文相同:
https://daveceddia.com/open-modal-in-react/
加上单击确认时的控制台日志记录.
如何实现该功能?
我需要使用 Redux 吗?或者是否有某种用于确认对话框的库?
我的代码:https://codesandbox.io/s/6n03myqw8w
这是一个没有使用 Redux 的例子.它展示了如何使用组件来解决必须依赖数据存储的问题.主容器保存数据和动作,我们只需传递这些动作和必要的数据即可删除和显示模式.
现在这不考虑加载和样式更改,但您可以将它们绑定到 MockModal 类中的操作以添加假加载图标(因为我们使用来自容器的数据,所以数据是即时的).样式更改可以添加到 MockModal 中的 removeTask
方法中,或者您可以研究一种动画技术来增强 UI.以下纯属函数式实现.
如果您需要更多帮助,请告诉我.
class MockModal extends React.Component {移除任务 = () =>this.props.removeTask(this.props.data);closeModal = () =>this.props.closeModal();使成为() {常量 { id, name } = this.props.data;如果(this.props.displayModal){返回 (<h5>你想删除任务 {id} : {name}</h5><button onClick={this.removeTask}>确认</button><button onClick={this.closeModal}>关闭</button></div>)}返回空值;}}类任务扩展 React.Component {showModal = (任务) =>this.props.show(this.props.task);使成为() {const { id, name } = this.props.task;返回 (<h5>{id}:{name}</h5><button onClick={this.showModal}>(删除)</button></div>)}}类 App 扩展 React.Component {状态 = {显示模式:假,模态:{身份证:空,名称:空,},任务: [{ id: 1, name: '星球大战' },{ id: 2, name: '哈利波特' },{ id: 3, name: '指环王' },],}showModal = (任务) =>this.setState({ modal: task, showModal: true, });hideModal = () =>this.setState({ showModal: false, });removeTask = (activeTask) =>{常量索引 = this.state.tasks.findIndex(任务 => {返回 task.id === activeTask.id;});这个.setState({显示模式:假,任务: [...this.state.tasks.slice(0, index),...this.state.tasks.slice(index + 1),]})}使成为(){返回 (<模拟模态displayModal={this.state.showModal}closeModal={this.hideModal}removeTask={this.removeTask}数据={this.state.modal}/>{this.state.tasks.map(task => {返回 (<任务键={task.id} 任务={task} 显示={this.showModal}/>)})}</div>)}}ReactDOM.render(<App/>, document.getElementById('root'));<script src="https://cdnjs.cloudflare.com/ajax/libs/react/15.1.0/react.min.js"></脚本><script src="https://cdnjs.cloudflare.com/ajax/libs/react/15.1.0/react-dom.min.js"></script><div id="root"></div>
I have an app which shows a list of items in rows. Every item has a corresponding delete button. When I click it, it shows a modal with confirmation. When I click 'cancel', the action is canceled. When I click 'confirm' the modal do as follows:
1. It displays a loading icon
2. It changes the style (color and text - now it says it is done)
3. It removes the item
What I get so far is just displaying modal, same as in this article:
https://daveceddia.com/open-modal-in-react/
Plus just console logging when confirm is clicked.
How do I implement the feature?
Do I need to use Redux? Or is there some kind of library for confirmation dialogs?
My code:
https://codesandbox.io/s/6n03myqw8w
解决方案 Here's an example without using Redux. It shows how to use components to get around the issue of having to rely on a data store. The main container holds the data and actions and we simply pass these actions and the necessary data to remove and display a modal.
Now this doesn't account for the loading and style changes but you can bind these to the actions within the MockModal class to add a fake loading icon (since we're using data from a container, the data is instant). The style change could be added to the removeTask
method in MockModal, or you can look into an animation technique to enhance the UI. The below is purely a functional implementation.
Let me know if you need more help.
class MockModal extends React.Component {
removeTask = () => this.props.removeTask(this.props.data);
closeModal = () => this.props.closeModal();
render() {
const { id, name } = this.props.data;
if (this.props.displayModal) {
return (
<div>
<h5>You want to delete task {id} : {name}</h5>
<button onClick={this.removeTask}>Confirm</button>
<button onClick={this.closeModal}>Close</button>
</div>
)
}
return null;
}
}
class Task extends React.Component {
showModal = (task) => this.props.show(this.props.task);
render() {
const { id, name } = this.props.task;
return (
<div>
<h5>{id}:{name}</h5>
<button onClick={this.showModal}>(REMOVE)</button>
</div>
)
}
}
class App extends React.Component {
state = {
showModal: false,
modal: {
id: null,
name: null,
},
tasks: [
{ id: 1, name: 'Star Wars' },
{ id: 2, name: 'Harry Potter' },
{ id: 3, name: 'Lord of the Rings' },
],
}
showModal = (task) => this.setState({ modal: task, showModal: true, });
hideModal = () => this.setState({ showModal: false, });
removeTask = (activeTask) => {
const index = this.state.tasks.findIndex(task => {
return task.id === activeTask.id;
});
this.setState({
showModal: false,
tasks: [
...this.state.tasks.slice(0, index),
...this.state.tasks.slice(index + 1),
]
})
}
render(){
return (
<div>
<MockModal
displayModal={this.state.showModal}
closeModal={this.hideModal}
removeTask={this.removeTask}
data={this.state.modal}
/>
{this.state.tasks.map(task => {
return (
<Task key={task.id} task={task} show={this.showModal} />
)
})}
</div>
)
}
}
ReactDOM.render(<App />, document.getElementById('root'));
<script src="https://cdnjs.cloudflare.com/ajax/libs/react/15.1.0/react.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/react/15.1.0/react-dom.min.js"></script>
<div id="root"></div>
这篇关于具有删除功能的反应模式的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持IT屋!
查看全文
登录 关闭
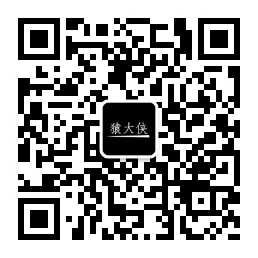